Table of contents
1. Abstract
2. Introduction
3. Coding Mathematics' problems
3.1. Inputs' wide possibilities
3.2. Agreement of input formulas with standard rules of math.
3.3. Length of input formula
4. Overview on the Implementation
4.1. Data class
4.2. MyChecker class
4.3. MyFixer class
4.4. Calculator class
5. How to use the implementation
6. Appendix
6.1. Linked lists
6.2. Bisection method
7. conclusion
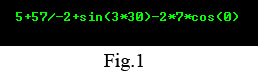
1. Abstract
Have you tried before to put the upper formula in one of your program, but you found its hard to calculate it? Have you stopped codding one of your programs because you need to treat with complex formulas and their isn't a route to calculate it in your programming language? have you gone to use programming language such as fortran or mathlab, because you need to use some of its mathematical power, as the same time if you have this power in your main language you may produce a better program? If you are one these slides I have told about, this article will be good for you inshallah as its give you two solutions to your problems; the first is how to program mathematical programs and the second a header file in c++ that you can use in order to calculate formula such as which in Fig.1, in order to facility your creative c++ program.
2. Introduction
Mathematical programming is the backbone of any programming language and the one of the most important reasons to invite computers and with subordination programming language, that is because all science in the world are depending on mathematics, so needing of easier routes to do it is continue one of the most required things in these days and will still obtainable until the end of the world, so from this point we are starting in order to implement a scientific calculator coded by myself me and will be coded by your self in the coming days inshallah, except you don't wanna.
In c++ there is some libraries work on mathematics such as math.h and c math, but these libraries don't give you all operations you may need, but at the same time they are the soul which we plant our implementation on.
3. Coding Mathematics' problems
During coding mathematical programs you will face some problems which have faced all programmers before, I put them under your hand in order to take attention, you may see this problems not a problem as the the word mean, but at all I write the problem which have faced me and caused me some difficulty during coding.
3.1. Inputs' wide possibilities
The first problem which may face you is the Input's wide possibilities, you don't know what the user will give you as inputs, so you should determine at first what kind of math your program will treat with, and make sure your user won't enter any thing outside it especially if you receive inputs as a string or stream, or you will receive it by a console interface, so to face this problem at first you should check the inputs and then do your maintenance progress on the input, for example:
- would your program maintain formulas with simple operators (+,-,/,*) only?
- or your program will go up until intersection operators with brackets ?
- or your program will go up until trigonometric functions (sin, cos, . . . ) ?
- and as you go, you should determine first the field you will depend on and then start.
3.2. Abbreviation or not substandard of input formulas
The second problem which may face you is user may enter input formulas with some abbreviation, such as 2x which means 2*x or 2(sin(45)) which means 2*sin(45)
or 2++++++x , but your program is coded to treat with one operator only, etc.
so you should edit your input formula first until it be adaptable to your program and then send it to your maintain function.
3.3. Length of input formula
The third problem which may face you is the inputs length, as the user may enter you short formula and may enter you a long one; In this condition you have two solutions: the first is using a long array which can read any length of the formula, but it's a bad solution from my opinion because it will waste memory if the user enter a small formula, The best solution from my opinion is using counters which depending on linked lists (see the appendix) as it is allocating memory as you need only, so you want allocate memory more than you need, In my case I use vectors .
4. Overview on the Implementation
4.1. Data class
Data
class is the container class of my implementation, in this class I put the main libraries which I need in the program and the public method which I will use as assistant functions as:
double charVtod (vectorch)
function convert a double number form the input formula to a double number
double getNum (vector &form, int &orderm, int &digit);
read a number form the input formula
unsigned getDigits(long num);
count the digits of a long number
vector dtoVchar (double num);
convert double numbers to a vector of characters
Collapse | Copy Code
class Data
{
protected:
int temp; char tempChar;
bool hasVar; double charVtod (vector<char>ch); double getNum (vector<char> &form, int &orderm, int &digit);
unsigned getDigits(long num);
vector<char> dtoVchar (double num);
};
4.2. MyChecker class
MyChecker
class is a class which using for checking the input formula and the agreement of it to my program mathematical cover as if the user enter a wrong formula; this class should alert him about that, wrong entry as such as:
x**x ,si(45), /52312, etc...
About functions:
inline bool isOp(char &ch);
is using for checking for normal operators +, -, *, /
b-bool isVar(vectorvars,char &ch);
is using for checking for variables
bool _check (vector &ch, vectorvars);
is the function which use other class function in order to check the entry formula
bool isTriangle (vector &ch , int &temp);
is the function which using for checking for trigonometric functions.
bool editSigns (vector &ch);
is the function which using to edit signs as ++ becomes + and -+ becomes -, etc.
bool check(vectorform, char mainVar );
is the function which using to check entry formula with one variable, read by the other argument mainVar
, such as 2x + 2 and mainVar
= 'x'
bool check(vectorform, vectorvars);
is the function which using to check entry formula with more than one variables read by the vector vars
such as 2x+3y
Collapse | Copy Code
class MyChecker : public Data
{
private:
inline bool isOp(char &ch);
bool isVar(vector<char>vars,char &ch);
bool _check (vector<char> &ch, vector<char>vars); protected:
bool isTriangle (vector<char> &ch , int &temp);
bool editSigns (vector<char> &ch);
bool check(vector<char>form, char mainVar ); bool check(vector<char>form, vector<char>vars); };
4.3. MyFixer class
MyFixer
class is the class which its minor is to edit formulas in order to make formula adaptable to my program and the next class calculator, for example: if the input is xx but * between them and the result become x*x; this function has only two functions; one of them is invoking the other:
void fix(vector &ch);
This function is invoked by other son classes and receive the formula and send it to the other function.
void _fix (vector &ch);
This function in invoked by the classes' function and do the required editing.
Collapse | Copy Code
class MyFixer : public MyChecker
{
protected:
void fix(vector<char> &ch);
private:
int temp;
void _fix (vector<char> &ch);
};
4.4. Calculator class
Calculator
class is the backbone of this program, this class's functions is the functions which give the calculation of a formula; with discovering its functions:
firstRank
, secondRank
and thirdRank
are functions which divide formula to three phases:
the first related to power and trigonometric functions, the second is for * and / operations and the third is for + and - operations, with putting on consider the function getBorder
is using for determinate the brackets which calculation is done withing and then delete it.
This class has 6 overloading of the function calc for working with the three cases which take characters vector, arrays and strings; the functions _calc
at final is the function which takes the formula from the six overloading functions and then do calculation.
Collapse | Copy Code
class Calculator : public MyFixer
{
private:
int str, end, digit,digit1,digit2, temp, temp1;
vector<char> charNum;
double num, num1, num2;
double getCaclNum (vector<char> &form, int &order, int &count);
void getBorder (vector<char> &form);
void firstRank (vector<char> &form); void secondRank (vector<char> &form); void thirdRank (vector<char> &form); vector<char> _calc (vector<char> formula);
vector<char> strToVector (string form);
vector<char> charToVector(char form [] );
public:
Calculator () :final(false){}
double calc (vector<char> formula);
double calc(char formula []);
double calc(string formula );
double calc (vector<char> formula, char var, double varValue);
double calc (char formula [], char var, double varValue);
double calc (string formula , char var, double varValue);
};
5. How to use the implementation
Now we have the part of how to use these classes:
- first you need to include these classes in your program
- make including in your cpp file for calculator.h file
- Make an object from calculator class
- use one of the six overloading
calc
functions
for example of you want to get a functions from the user with one variable, then get the variable's value and print the result, the code will be as the following:
Collapse | Copy Code
#include "Calculator.h"
#include <string>
using namespace std;
void main ()
{
Calculator c1; string form; char var; double value; while(true)
{
cout<<"Please, enter your formula:";cin>>form;
cout<<"enter your variable:";cin>>var;
cout<<"Enter "<<var<<" value:";cin>>value;
cout<<"result = "<'